You are viewing a submission by a user. This is the read only version. Try solving this
question here.Advanced Code Challenges
Intermediate to Advanced programming challenges for next level preparation.
Implement a function to read a field inside a nested object | AWS Frontend Interview Question | JavaScript Interview Question
Submission by
@Yomesh Gupta
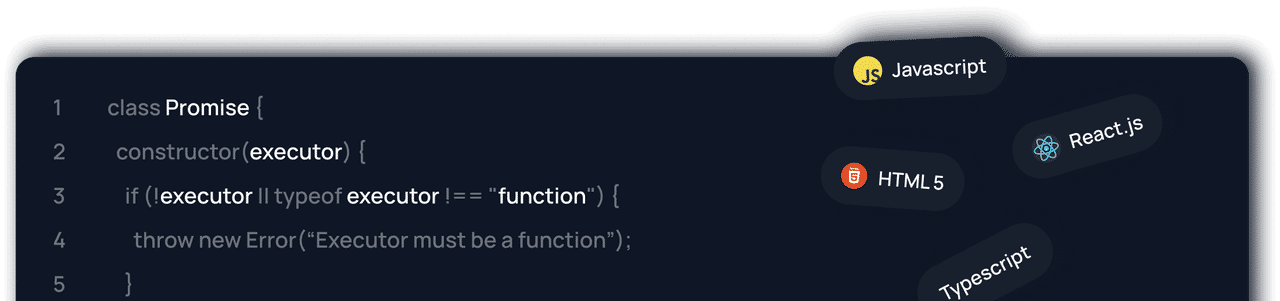
Start solving today!
For Free
Log in or sign up to view this question. Join now to access exclusive content and get full access.
- Access to 200+ questions.
- Access to our solutions and videos.
- Ability to run code and test your solution.
- Track your progress and see your submissions.
Premium Frontend Tutorials
For Free
Intermediate to Advanced programming content that helps you understand what happens under the hood and makes it easy for you to grow in your career.
Watch Now
https://www.devtools.tech/